Welcome to the Trimester3DataScurtures wiki!
Notes and Takeaways
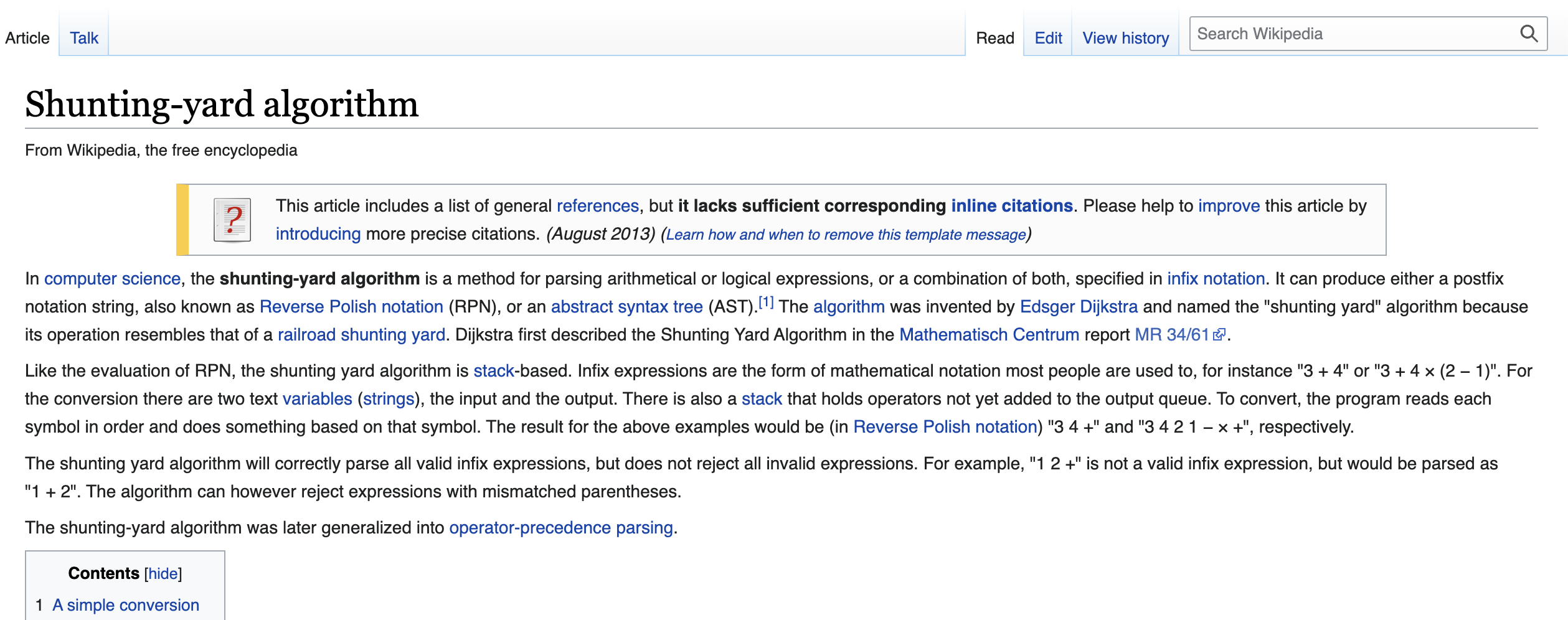
- This was an algorthim that I researched about online, with my scrum team and we were able to use this alogrithim to help pave us and give us to use for the square root function of our calculator, which was really awesome and cool to find!
- In mathematics, an expression or mathematical expression is a finite combination of symbols that is well-formed according to rules that depend on the context.
In computers, expression can be hard to calculate with precedence rules. In computer math we often convert strings into Reverse Polish Notation (RPN, 3 + 4 becomes 3 4 +) using the Shunting-yard algorithm. Review Wikipedia diagram and the code and you will see the need for a Stack.
Plans and requirements for Week 2
- GitHub review page that documents key learnings using code snippets
- Calculator Code with SQRT function, Replit Runtime, with runnable (no input) Test Data, and input option
Notes and Takeaways: Week 1
- Linked list are a way of keeping and managing a list of Objects
- Different objects are assigned different nodes and each node leads to the next node in line. Starting from the head and pointing toiwards the next node and thus, iritiating through the list and getting the output that you want through a variation of the code
- Some examples are deleting a specific node, reversing the list or even try and make it do a weird pattern
- Queues can be built using Linked List Objects
- A Queue requires keeping track of First In for dequeue extraction (head node)
- A Queue requires keeping track of the Back for enqueue entry (tail node)
- A Queue requires keeping track of the Current node for iteration (current node)
- First in, is the first object/node that is going to be the first out
- Stacks can be built using Linked List Objects
- A Stack requires keeping track of Last Item inserted to support Push entry and Pop extraction (lifo node) Last in, first out
- From what I can see, it is clear that stacks and queuesd are oppsote from each other in which how the obejcts and nodes interact weith one another and thier main functions
Plans and requirements for Week 1
- GitHub review page that documents key learnings using code snippets,
- GitHub review page, Replit for runtime to TT1 Challenges.
- Describe Linked Lists, Describe Java Generic T
- Queue Add and Delete
- Merge 2 Queues
- Build Stack and reverse Queue Order
Notes and Takeaways
- Data structures are used to organize data which are more sequences or tables of values rather than a single value
An example of this is a list of numbers
- There are different types of data structures which are primitive and non-primitive
- Algorithms interact with data structures to create optimized code
- Imperative programming uses statements to change a program’s states
- Objected Oriented Programming uses classes and objects to create objects and instances of them -> constructors, encapsulation, abstraction, polymorphism
Plans and requirements for Week 0
- Create an alternative menu in Java with a data structure and try and catch which is dynamic, rather than simply having switch statements that are hardcoded. This will likely use abstraction because subclasses are needed.
- Create a java class that can swap two numbers based on value. It prints the numbers first in the order given, then switches them and organizes them by value -> prints this out.
- Use 2D arrays in Jaca to print a formatted matrix; this has a toString method to format and output a String and properly accessing values in the 2D Array